Build your own code for Fast Fourrier Transform (FFT)
What is the FFT ?
The Fast Fourier Transform (FFT) is a highly efficient algorithm for computing the Discrete Fourier Transform (DFT) and its inverse. The DFT transforms a sequence of complex numbers, typically representing time-domain signals, into a sequence of complex numbers representing the frequency domain. The FFT dramatically reduces computation time compared to directly calculating the DFT, making it essential for real-time signal processing applications such as audio and image processing, telecommunications, and data compression.
The Fast Fourier Transform (FFT) is a powerful algorithm that significantly reduces the complexity of computing the Discrete Fourier Transform (DFT) from [math]O(N^2) to O(N log( N))[/math], where N represents the number of data points. This efficiency makes FFT a cornerstone in digital signal processing (DSP), widely used for tasks such as filtering, spectral analysis, and solving partial differential equations. Various FFT algorithms exist, with the Cooley-Tukey algorithm being the most prevalent, alongside other specialized algorithms tailored for different data structures and specific requirements. By converting signals into the frequency domain, FFT allows for more straightforward analysis and manipulation of frequency components, enhancing the ability to process and interpret complex signals.
Why we use it ?
The Fast Fourier Transform (FFT) is used in a wide range of applications due to its ability to efficiently transform signals between the time domain and the frequency domain. Here are some of the primary uses of FFT:
- Signal Processing:
- Filtering: FFT is used to design and implement filters that can remove noise or extract specific frequency components from a signal.
- Spectral Analysis: By analyzing the frequency spectrum of a signal, FFT helps in identifying the presence and strength of various frequency components.
- Communications:
- Modulation and Demodulation: FFT is used in digital communication systems for modulating and demodulating signals.
- OFDM: Orthogonal Frequency-Division Multiplexing (OFDM), a widely used technique in broadband communications, relies on FFT for efficient modulation and demodulation.
- Audio Processing:
- Compression: Audio compression algorithms like MP3 and AAC use FFT to transform audio signals into the frequency domain for more efficient encoding.
- Equalization: FFT allows for the adjustment of specific frequency bands in audio signals to enhance or suppress certain sounds.
- Image Processing:
- Image Compression: Algorithms such as JPEG use FFT to transform images into the frequency domain for more efficient compression.
- Filtering: FFT is used for image filtering operations, such as blurring, sharpening, and edge detection.
- Biomedical Applications:
- ECG and EEG Analysis: FFT is used to analyze the frequency components of electrocardiogram (ECG) and electroencephalogram (EEG) signals for medical diagnostics.
- Radar and Sonar:
- Signal Analysis: FFT helps in analyzing the reflected signals in radar and sonar systems to determine the distance and speed of objects.
- Vibration Analysis:
- Mechanical and Structural Analysis: FFT is used to analyze the vibration characteristics of machinery and structures to detect faults or predict failures.
- Astronomy:
- Signal Detection: FFT is used in radio astronomy to analyze signals from space, helping in the detection of celestial phenomena.
- Speech Recognition:
- Feature Extraction: FFT is used to extract features from speech signals, which are then used in speech recognition algorithms.
- Scientific Research:
- Numerical Solutions: FFT is used to solve partial differential equations and perform other numerical computations in various scientific fields.
Overall, FFT is a versatile and essential tool in any field that involves signal processing and analysis, providing a fast and efficient way to work with frequency-domain representations of signals.
How to compute it?
Let’s consider a discrete signal E(k) of N points. The Fast Fourier Transform (FFT) of this signal, represented as a vector FFT_E of the same size, is computed by performing matrix multiplication using the following formula:
[math]
FFT\_E(k) = \frac{1}{\sqrt{N}} \mathbf{W}_N = \frac{1}{\sqrt{N}}
\begin{bmatrix}
1 & 1 & 1 & \cdots & 1 \\
1 & \omega & \omega^2 & \cdots & \omega^{N-1} \\
1 & \omega^2 & \omega^4 & \cdots & \omega^{2(N-1)} \\
\vdots & \vdots & \vdots & \ddots & \vdots \\
1 & \omega^{N-1} & \omega^{2(N-1)} & \cdots & \omega^{(N-1)(N-1)}
\end{bmatrix}
[/math] where [math]\omega = e^{-j \frac{2 pi}{N}}[/math] with [math]j^2=-1[/math]
As exemple:
- For 2 points [math]\omega_2 = e^{-j\frac{2\pi}{2}} = -1[/math] so [math]\mathbf{W} = \frac{1}{\sqrt{2}}
\begin{bmatrix}
1 & 1 \\
1 & -1
\end{bmatrix}
[/math] - For 4 points [math]\omega_4 = e^{-j\frac{2\pi}{4}} = -j [/math] so [math]\mathbf{W} = \frac{1}{2}
\begin{bmatrix}
1 & 1 & 1 & 1 \\
1 & -j & -1 & j \\
1 & -1 & 1 & -1 \\
1 & j & -1 & -j
\end{bmatrix}[/math]
Python Code
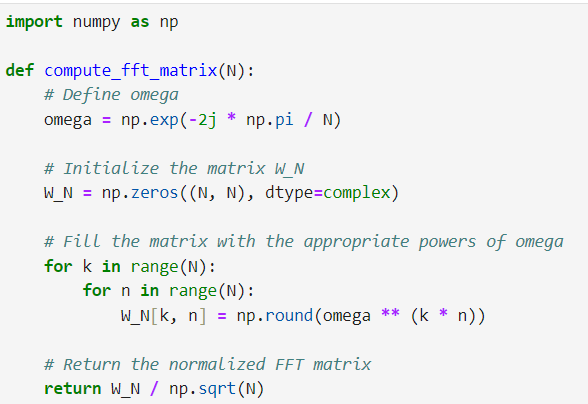
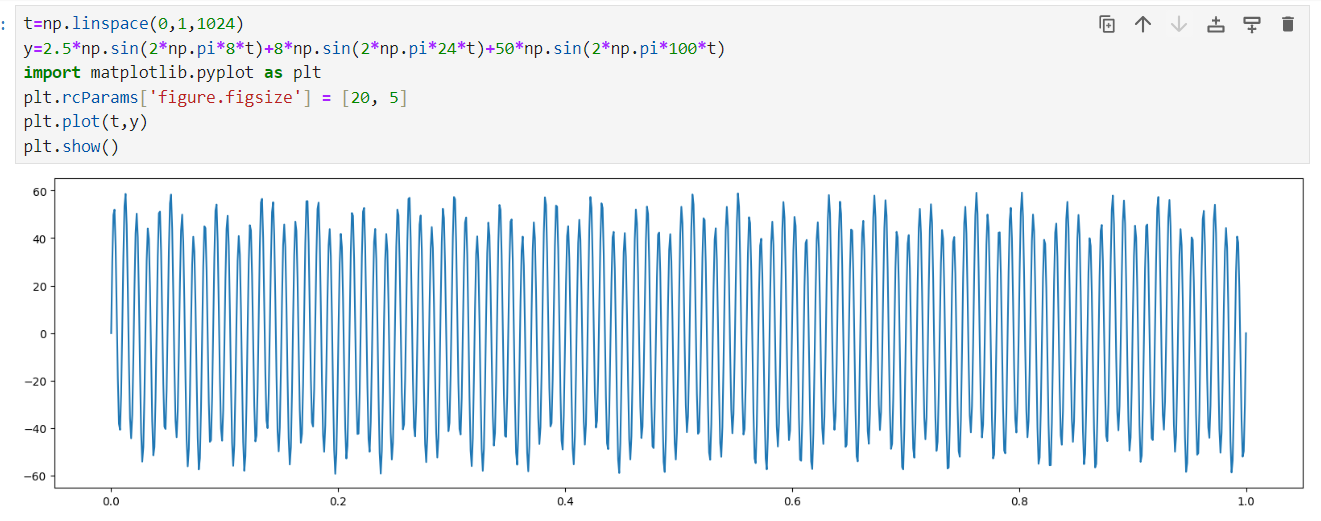
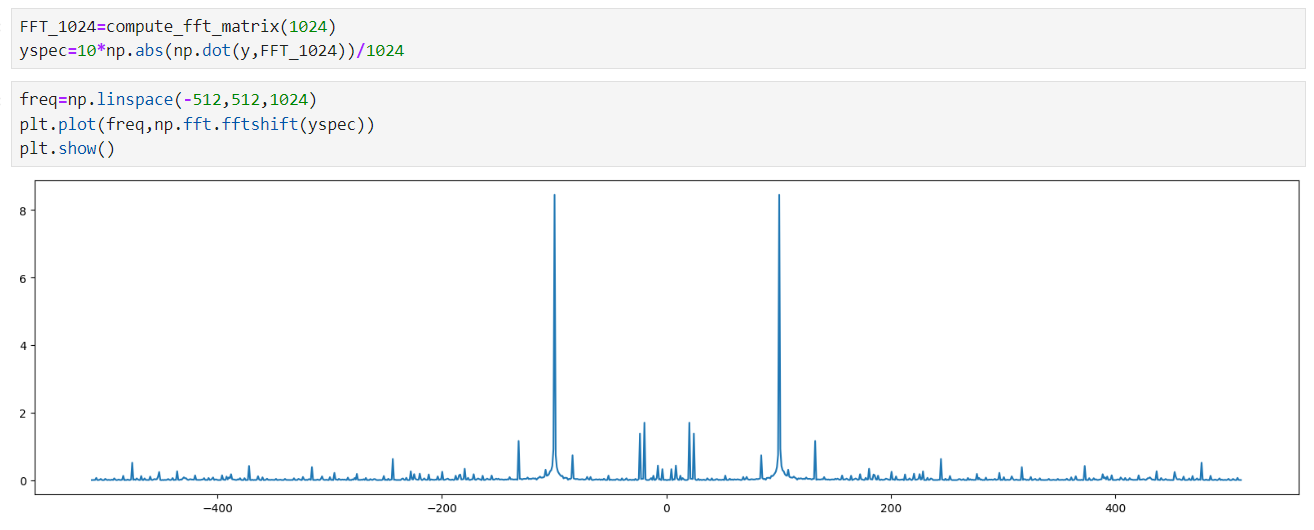